Okay, so today I wanted to mess around with printing the top processes on my Linux machine, kind of like what the top command does, but maybe with a bit more control. I named it “leo print top” just for kicks, you know, a simple, memorable name.
First Steps
First off, I needed to figure out where Linux keeps all this process info. Turns out, it’s all in the /proc directory. Each process has its own folder in there, named after its process ID (PID). Inside each folder, there’s a bunch of files with all sorts of details about the process.
Getting the PIDs
So, my first move was to write a little Python script to grab all the PIDs. I used the function to get a list of all the entries in /proc. Then, I filtered out the ones that were actually directories and whose names were numbers (those are the PIDs).
Here’s a snippet of what that looked like:
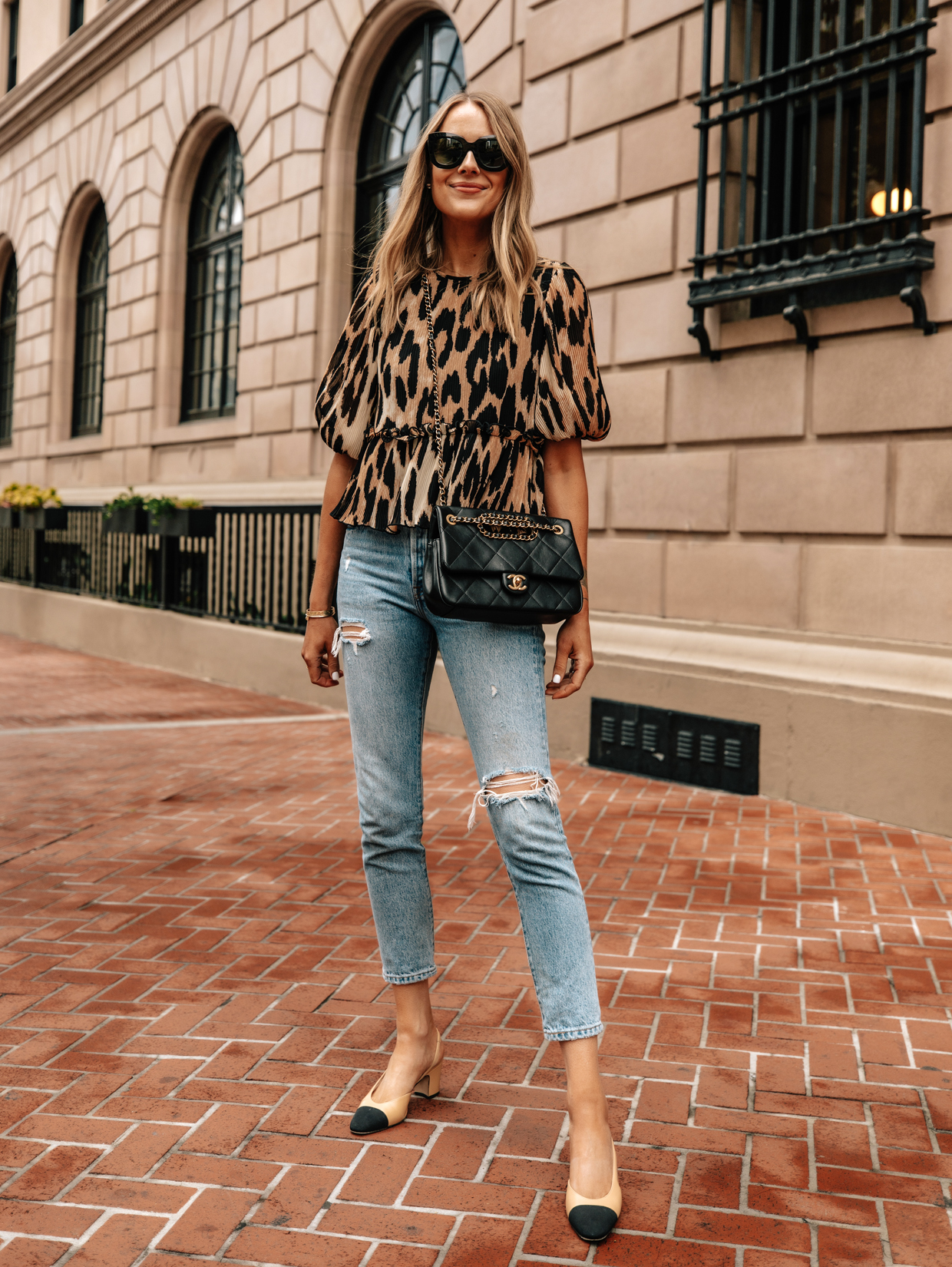
import os
pids = [pid for pid in *('/proc') if *() and *(*('/proc', pid))]
Reading Process Info
Next up, I needed to read the info for each process. The stat file in each process directory has most of what I needed. It’s a single line with a bunch of fields separated by spaces. I wrote a function to open this file, read the line, and split it into a list.
Here’s how I did it:
def read_stat(pid):
with open(*('/proc', pid, 'stat'), 'r') as f:
return *().split()
Parsing the Data
Now, this stat file is a bit cryptic. The fields aren’t labeled, you just gotta know what they are by their position. I looked up the format and picked out the fields I was interested in, like the process name, state, and CPU time.
Calculating CPU Usage
To get the CPU usage, it’s not as simple as reading a single value. You have to read the CPU time, wait a bit, read it again, and then calculate the difference. Then, divide that by the time interval you waited. I added some code to do this, storing the previous CPU time and timestamp for each process.
Sorting and Displaying
After getting all this data, I sorted the processes by CPU usage. Python’s sorted() function with a custom key made this easy. Then, I just printed out the top few processes in a nice, readable format.
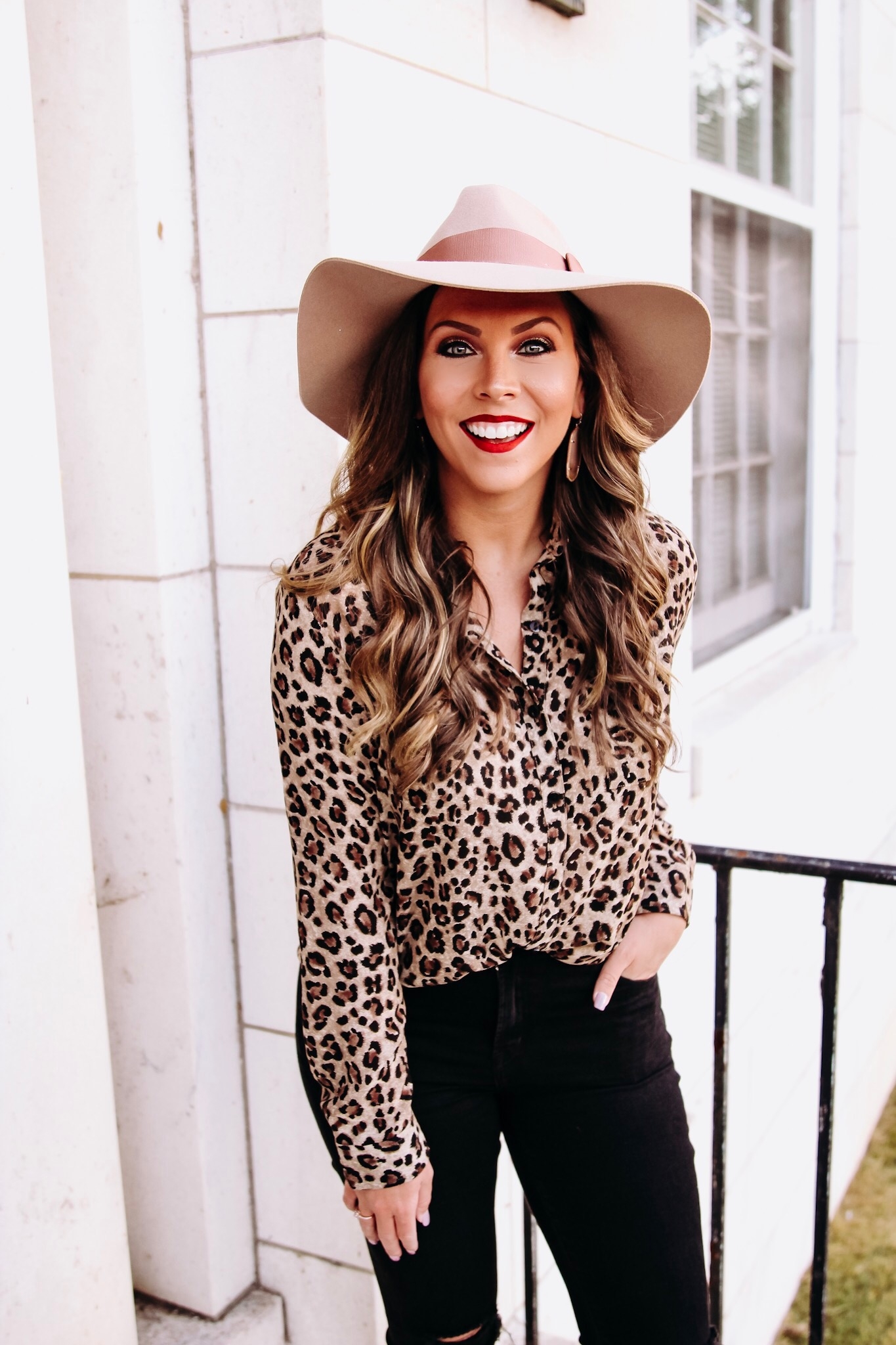
Final Touches
I threw in some error handling, like checking if a process disappeared while I was reading its info. Added a loop to keep updating the display every few seconds. It was pretty cool to see it all come together, a personalized “top” command, made from scratch!
And there you have it! That’s how I spent my day, tinkering with process info and Python. “leo print top” might not be the fanciest tool, but it was a fun little project, and I learned a ton about how Linux processes work under the hood. Hope you enjoyed this little walkthrough!